Update a file through a Python algorithm
Project description
As part of my role, I am required to regularly update a file that identifies the employees who can access restricted content.
The contents of the file are based on who is working with personal patient records. Employees are restricted access based on their IP address.
There is an allow list for IP addresses permitted to sign into the restricted subnetwork.
There's also a remove list that identifies which employees you must remove from this allow list.
My task is to create an algorithm that uses Python code to check whether the allow list contains any IP addresses identified on the remove list. If so, the algorithm should remove those IP addresses from the file containing the allow list.
Assigning the "allow" and "remove" list to variables
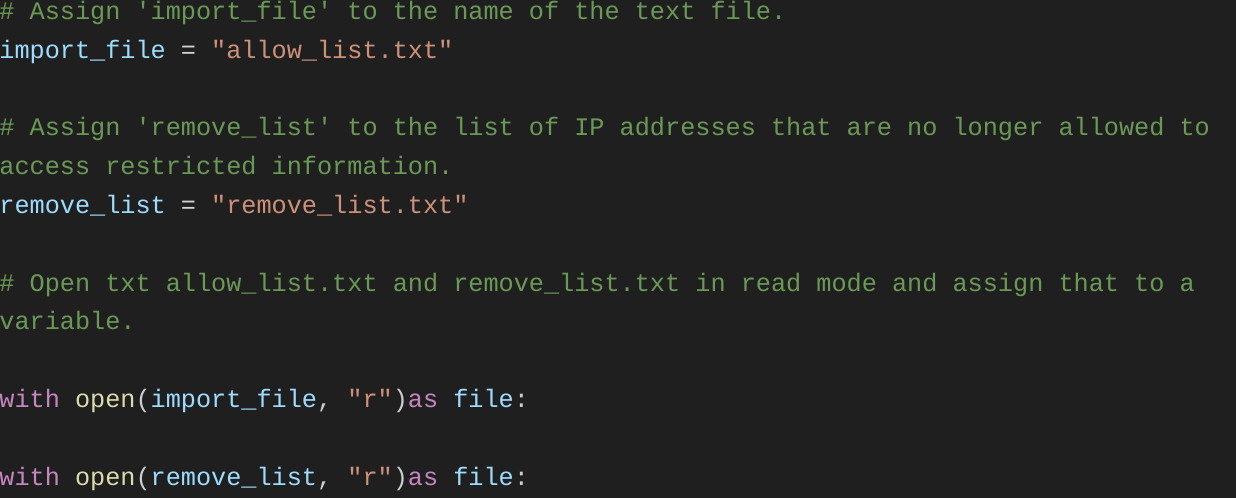
Code block:
import_file = "allow_list.txt"
remove_list = "remove_list.txt"
with open(import_file, "r")as file:
with open(remove_list, "r")as file:
Code review:
In my algorithm, the
Read the files content

Code block:
with open(import_file, "r")as file:
allow_ips = file.read()
with open(remove_list, "r")as file:
remove_ips = file.read()
Code review:
While utilizing the
Convert the string into a list
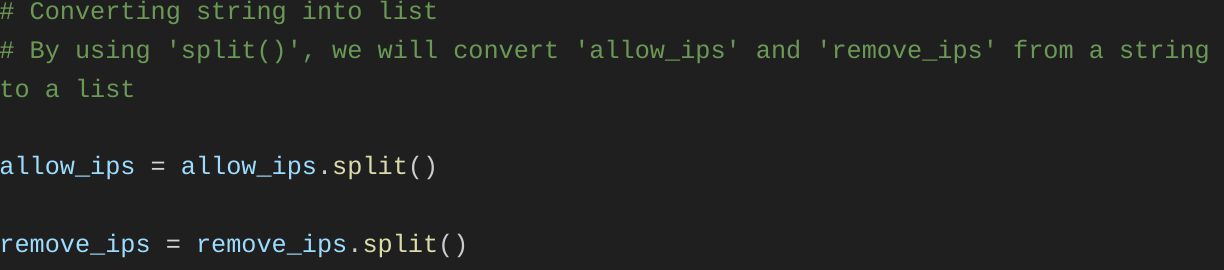
Code block:
allow_ips = allow_ips.split()
remove_ips = remove_ips.split()
Code review:
In order to remove individual IP addresses from the allow list, I needed it to be in list format. Therefore, I next used the
Iterate through the remove list

Code block:
for element in allow_ips:
Code review:
To facilitate a crucial component of my algorithm, I implemented an iterative process that involves cycling through the IP address enlisted in the
In Python, the
Remove IP addresses that are on the remove list
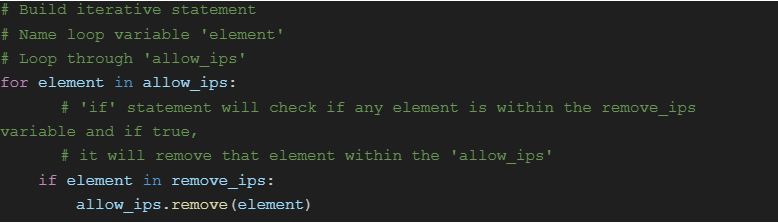
Code block:
for element in allow_ips:
if element in remove_ips:
allow_ips.remove(element)
Code review:
To implement my algorithm, it was necessary to eliminate any IP address from the allow list, named
Initially, in my for loop, I established a condition to check if the loop variable element was present in the
Subsequently, within that condition, I utilized
Update the file with the revised list of IP addresses

Code block:
allow_ips = "\n".join(allow_ips)
Code review:
For the last stage of my algorithm, I had to revise the list of IP addresses in the allow list file. To achieve this, I began by coverting the list back into a string. I utilized the
The
Subsequently, I implemented another with statement along with the

Code block:
with open(import_file, 'w') as file:
file.write(allow_ips)
Code review:
This time, I added a second argument,
In this scenario, my aim was to write the updated allow list as a string to the
Summary
I devised an algorithm to eliminate IP addresses from the
Next, I iterated throught the IP addresses in